Python Program of Basic Questions (Part-2)
The following Python section contains a wide collection of Basic Python programming examples. This is Python Program of Basic Questions (Part-2), another part-1 is in another section.
1. Write a Python program to print the following string in a specific format.
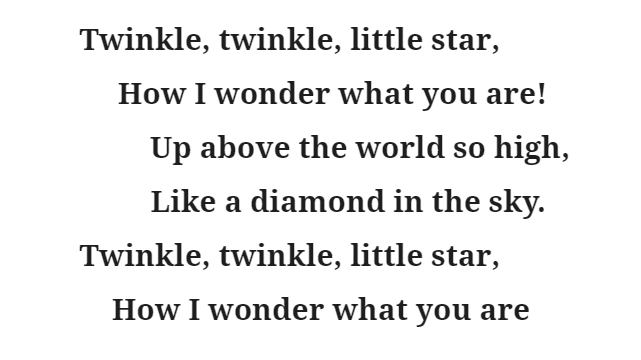
p='Twinkle, twinkle, little star,\n How I wonder what you are!\n Up above the world so high,\n Like a diamond in the sky.\nTwinkle, twinkle, little star,\n How I wonder what you are' print(p)
Output:
Twinkle, twinkle, little star,
How I wonder what you are!
Up above the world so high,
Like a diamond in the sky.
Twinkle, twinkle, little star,
How I wonder what you are
2. Write a Python program that read a string from a user and print the sting in double quotation marks.
str=input("Enter a string= ") print('""',str,'""')
Output:
Enter a string= I Am Coding Lover
“” I Am Coding Lover “”
3. Write a Python program which accepts the radius of a circle from the user and compute the area.
r=int(input("Enter radius of circle= ")) area= 22/7*(r*r) print("Area of Circle= ", area, "sq")
Output:
Enter radius of circle= 6
Area of Circle= 113.14285714285714 sq
4. Write a Python program that will accept the base and height of a triangle and compute the area.
b=int(input("Enter base of triangle= ")) h=int(input("Enter height of triangle= ")) area= 1/2*b*h print("Area of Triangle= ", area)
Output:
Enter base of triangle= 4
Enter height of triangle= 6
Area of Triangle= 12.0
5. Write a Python program to perform swapping of two numbers with or without third variable.
a=int(input("Enter a value of A= ")) b=int(input("Enter a value of B= ")) a=a+b b=a-b a=a-b print("Value of A= ", a, "\nValue of B= ",b)
Output:
Enter a value of A= 55
Enter a value of B= 99
Value of A= 99
Value of B= 55
6. Write a Python program that read a 3 digit number from user and perform the addition of their digits.
a=int(input("Enter a 3 Digit Number = ")) if a>100 and a<999: x=a//100 y=(a-x*100)//10 z=a-x*100-y*10 print("Sum of Your 3 Digit Number= ", x+y+z) else: print("SORRY!!! Please Enter Only 3 Digit Number")
Output:
Enter a 3 Digit Number = 578
Sum of Your 3 Digit Number= 20
7. Write a python program to find the greatest number among three.
a=int(input("Enter a Number = ")) b=int(input("Enter a 2nd Number = ")) c=int(input("Enter a 3rd Number = ")) if a>b and a>c: print(a," is greatest number") elif b>a and b>c: print(b," is greatest number") else: print(c, " is greatest number")
Output:
Enter a Number = 777
Enter a 2nd Number = 950
Enter a 3rd Number = 586
950 is greatest number
8. Write a python program to display a greet message according to the marks obtained by the student.
a=int(input("Enter Your Marks in % = ")) if a>=90 and a<=100: print("Excellent") elif a>=70 and a<=90: print("Very Good") elif a>=60 and a<=70: print("Good") elif a>=40 and a<=60: print("Hard Work Needed") elif a>=0 and a<=40: print("Better Luck Next Time") else: print("SORRY!!! Please Inter Valid Marks in %")
Output:
Enter Your Marks in % = 90
Excellent
9. Enter basic salary from the user. Write a program to calculate DA and HRA on the following conditions:-
Salary DA HRA
<=2000 10% 20%
>2000 && <=5000 20% 30%
>5000 && <=10000 30% 40%
>10000 50% 50%
a=int(input("Enter Your Salary = ")) if a<=2000: da=a*10/100 HRA=a*20/100 print("Your DA= ", da) print("Your HRA= ", HRA) elif a>2000 and a<=5000: da = a*20/100 HRA = a * 30 / 100 print("Your DA= ", da) print("Your HRA= ", HRA) elif a>5000 and a<=10000: da = a * 30 / 100 HRA = a * 40 / 100 print("Your DA= ", da) print("Your HRA= ", HRA) elif a>10000: da = a * 50 / 100 HRA = a * 50 / 100 print("Your DA= ", da) print("Your HRA= ", HRA)
Output:
Enter Your Salary = 100000
Your DA= 50000.0
Your HRA= 50000.0
I Think You Understand above Topic “ Python Program of Basic Questions (Part-2) ”.
For any query or suggestions feel free to fill comment section and send it.
More Topic of Python:
Python Program of Basic Question’s Part-1
Top Python Program of String for beginner
Mind Blowing While Loop program for beginner
Recent Comments